
In this tutorial, you will build a simple upload form and save the uploaded photo in the storage.
File Upload
Uploading photos and documents is one of the most common use-cases in web development. If you’re just started, you learn form creation, form post, following with file upload. If you’re familiar with the form, Laravel saves your time from repeating the code of moving the uploaded file from temp folder to application folder.
First of all, bootstrap the project.
Create a new project
// Terminal
composer create-project --prefer-dist laravel/laravel photo-management
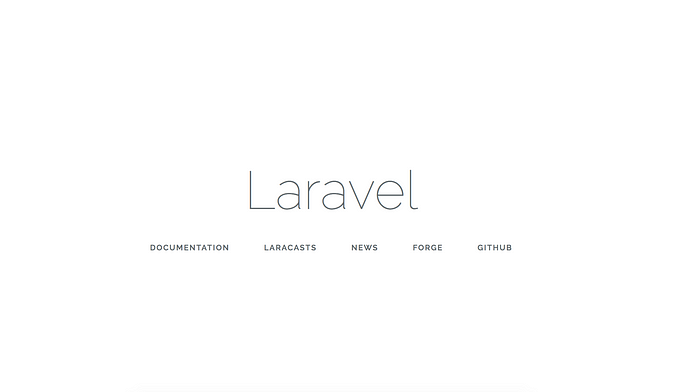
Let’s get started.
Create resource controller
Laravel provides a handy command to generate a CRUD controller.
// Terminal
php artisan make:controller PhotoController --resource
Controller created successfully.
The built-in welcome page is meaningless here. Remove the default route and register a resourceful route to the controller.
// routes/web.php
Route::resource('/', 'PhotoController’);
Next, craft the user interface.
Craft user interface
You create a layout file which is used across the application.
// resources/views/layouts/app.blade.php
<!doctype html>
<html lang="{{ app()->getLocale() }}">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Photo Management</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta/css/bootstrap.min.css"; integrity="sha384-/Y6pD6FV/Vv2HJnA6t+vslU6fwYXjCFtcEpHbNJ0lyAFsXTsjBbfaDjzALeQsN6M" crossorigin="anonymous">
<!-- Styles -->
<style>
body {
height: 100vh;
}
</style>
</head>
<body>
<div class="d-flex justify-content-center align-items-center" style="height: 100%">
@yield('content')
</div>
</body>
</html>
Create a blade file which is extending the layout file. In the blade file, you add a form with a file input and a submit button.
// resources/views/index.blade.php
@extends('layouts.app')
@section('content')
<form>
{{ csrf_field() }}
<div class="form-group">
<label class="custom-file">
<input type="file" name="photo" class="custom-file-input">
<span class="custom-file-control"></span>
</label>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
@endsection
Create a blade file for displaying a success message.
// resources/views/success.blade.php
@extends('layouts.app')
@section('content')
<h2>Upload succeed!</h2>
@endsection
Then, setup the upload form.
Setup upload form
In a normal form, form submission is transferring string like value to the server. The photo is not a string like value. With the enctype attribute, you can encode the photo when submitting to the server.
// resources/views/index.blade.php
<form method="POST" action="/" enctype="multipart/form-data”>
…
</form>

After submitting the form, you retrieve the uploaded photo.
Retrieve uploaded photo
You can access to the uploaded photo from the file method in Illuminate\Http\Request.
// app/Http/Controllers/PhotoController.php
public function store(Request $request)
{
$path = $request->file('photo')->store('images');
return view('success');
}
Retrieve the uploaded file and store into the folder named images in the storage folder.

By default, the store method will generate a unique ID to serve as the file name. Don’t worry. You can always store with your desired file name.
Store with custom file name
Use storeAs method, it accepts the second argument as the custom file name. You combine the file name with the extension. If not, the photo will store without any extension.
// app/Http/Controllers/PhotoController.php
public function store(Request $request)
{
$extension = $request->file('photo')->extension();
$path = $request->file('photo')->storeAs('images', 'my_photo.' . $extension);
return view('success');
}

What’s next
Next tutorial will be talking about validating the uploading file.
If you haven’t subscribed to my email list on the homepage, do it now. I’ll notify you when the new tutorial is published.
Did I tell you that you’ll receive a series of FREE tutorials when you subscribe? Go check it out.
Stay tuned.
Originally published at I Teach You How To Code.